Introduction
Web Development is the production of applications and documents that live primarily on the web, mainly interacted with via the web browser. The predominant tools involved are HTML (HyperText Markup Language), CSS (Cascading Style Sheets), and JavaScript (also known as ECMAScript). While there are often other tools involved depending on the specific needs and complexities of each application/web page, the common theme is that these three tools are the foundation on which nearly all user facing web pages are built on. Over the course of this and the next two articles, I will do my best to give a general overview and introduction to each of these tools, starting with HTML.
How I Got Started
I know that when I first started to learn the basics of Web Development only a short two and a half years ago, I found learning HTML to be the most boring aspect. Aside from some short tutorials around making basic websites on youtube, I had actually already begun programming a basic todo list using NodeJS. This application's end result was a CLI interface, meaning that there was no interaction with the browser, and rather, utilized NodeJS's REPL to create, read, and update a basic todo list via the terminal.
Rather than starting off with trying to create a visually appealing website, my mentor (who had assigned me this as my first programming project) instead had me start my programming journey writing a todo list without any "Front End" to speak of. I actually thought I never committed this very first project to Github, but in reviewing material for this blog post, it became apparent that indeed I had.
Most self taught developers first learning to code would not be instructed to create a CLI todo list as their first coding project, but my mentor was never one to shy away from difficult projects and still to this day knows exactly how far into the deep end to throw me (just enough to make me feel uncomfortable, but not enough to feel overwhelmed). Please note that this first project is fraught with poor decisions and is not a good reference on how to create a todo app. Outside of tutorials that essentially held my hand through the process (i.e. Free Code Camp), I had not really thought much on HTML, let alone CSS. Instead server side JavaScript was my initial introduction, and I had never played much in what is commonly known as "The Front End".
The Front End Vs The Back End
When reading about the "Front End" in regards to web development, many make the correlative analogy to a restaurant when trying to explain how a basic web application works. This is an over simplification, but is nevertheless useful as an explanation for beginners, and it goes something like this:
From your desktop computer, laptop computer, tablet, and/or mobile device, when you interact with any application that requires access to the internet; generally speaking this means that that device is interacting with another computer over a series of wires, wifi, or bluetooth. Your device, commonly referred to as a "Client" under these circumstances, makes a "request" (specifically what is known as a "GET request") for data from another computer somewhere else in the world. This other computer, commonly known as a "Server", receives the request, processes it, and if it has the desired data, will generally provide that data back over the internet to the "Client".
Given this simple explanation, hopefully one can see how this is like a waiter at a restaurant. You, the client, head into the restaurant (log onto the internet), and browse the menu ("The Front End", i.e. use the web browser via the url bar or search engine). You eventually see a meal (data) that you wish to eat (have access to). You make a request to the waiter (your internet service provider) for which item of food (data) you wish to eat (have access to). The waiter (your internet service provider) then proceeds (transmits the request over the internet) to the kitchen ("The Back End"). The waiter then relays your request to the kitchen staff (the server), who then proceed to prepare your dish utilizing ingredients (data) and a recipe (business logic). Once prepared, the waiter (your internet service provider), then returns with your meal (your requested data), which you then proceed to consume (either by reading an article, looking at pictures, taking care of work related business, etc.).
This relationship between a Client and a Server is an essential piece of knowledge that you will undoubtedly revisit many times over the course of your learning journey/career. Indeed this abstraction is not only the domain of the web, but is useful when thinking about processes that take place even within the confines of the hardware on your devices. I will, for the sake of simplicity, digress on this point in the interest of moving onto our main topic, HTML.
The HyperText Markup Language
The HyperText Markup Language, colloquially known as "HTML", has a long history dating back to the early days of the internet in the 1980s. Please take note that HTML is not a Programming Language, but rather is a Markup Language, which utilizes a series of recognized textual patterns and/or symbols within a text document to control the structure and formatting of said document. This establishes a recognizable relationship of various visual elements (text, images, videos, etc.) within the document, commonly known as a "Page", and more specific to HTML, a "Web Page".
These elements create a structure which the Web Browser (Chrome, Firefox, Edge, Safari, etc.) can parse through and format appropriately. HTML by itself can generate very basic documents and presentations on the web, but usually needs CSS (Cascading Style Sheets) to color and stylelize the elements, and oftentimes the JavaScript programming language is utilized to create interactivity with these elements. The following two articles will give you a basic introduction to CSS and JS (JavaScript), but it is important to note that without understanding the basics of HTML, one will not get far when researching CSS and JS, as both CSS and JS are meant to work with HTML as a part of a "stack" of programming tools to create a fully functioning web page.
Diving In, HTML Basics
To hammer home the fact that the Web Browser will attempt to interpret whatever textual document you give it into an HTML page. Let's start by writing up a simple txt file and seeing what happens when we open it in the browser. In previous articles, I have written about how working from the Command Line is a worthy pursuit of your time to learn. Thusly, while you can indeed make directories, and create files from a traditional file manager, in the following tutorials we will be using the command line and a basic shell (bash or zsh will be fine), to demonstrate.
Within your terminal make a new directory called "my_webpage" and navigate into it:
#bash shell
[ ~]$ mkdir my_webpage && cd my_webpage
From here you'll want to create a simple .txt file:
#bash shell
[ my_webpage]$ touch hello_world.txt
Using your favorite code editor open this up, most will probably use VSCode so:
#bash shell
[ my_webpage]$ code hello_world.txt
Simply type in a basic "Hello World!" example:
#hello_world.txt
Hello World!
Exit your text editor and now open your new file using your favorite browser:
#bash shell
[ my_webpage]$ firefox hello_world.txt
As you will soon see, the txt file has been rendered, displaying an extremely basic printing of your text in the browser:
Now on its own, this is rather underwhelming. The text is small and while possible, the browser is doing some work to parse out exactly what we're trying to have it render. Let's learn our first HTML tag, the <h1>
tag:
HTML Tags
The general demarcation characters utilized primarily in HTML are the "lesser than", "<", and the "greater than", ">", characters. These characters are used to enclose an html element. After writing this "opening tag", we then input whatever text we wish to be encapsulated within said tag and provide a "closing tag". The closing tag is quite similar to the opening tag, except a forward slash character, "/" is used to demarcate that this is meant to be the end of the HTML element. A simple example would be to enclose a h1, (a primary heading tag) inside of the "<" and the ">". Let's rename our "hello_world.txt" to "hello_world.html" using the "mv
" command:
#bash shell
[ my_webpage]$ mv hello_world.txt hello_world.html
This will rename our hello_world file to the appropriate extension, and furthermore, will enable better syntax highlighting in our editor. Giving our file the ".html" extension will also make it clear to ourselves (as well as others) what kind of file this is. Now within our hello_world.html file, let's enclose our text, "Hello World!", in html h1 tags like so:
<!--hello_world.html-->
<h1>Hello World!</h1>
If we now open up this file in our browser, we'll notice that the formatting of our text is resized to reflect this new assignment of the text as a heading:
HTML Tags, also called HTML Elements, are utilized by the browser to interpret how the developer wishes to organize the text, images, videos, and other forms of media in the HTML document. Additionally, HTML tags can be referenced directly within CSS and JavaScript files for further styling and programming interactivity with those elements. These elements can be standalone entities, or can contain other HTML elements within them. These are often referred to as "child," or "nested" HTML elements.
Nested HTML
Very much like a document written in a word processor (be it an essay, formal letter, or some other textual presentation), an HTML document follows certain protocols and best practices. Unlike more user facing textual documents written in a word processor, however, HTML documents are not only meant to be read by humans, but also parsed and interpretted by the Web Browser. By appropriately demarcating our HTML tags with the "<" and ">" symbols mentioned earlier, we are taking our first steps towards understanding this paradigm under which the Web Browser parses through an HTML document. These symbols essentially tell the Browser "this is an h1 tag, please make it bold and increase the font size." (This is an oversimplification, but is a satisfactory explanation for the purposes of introduction to the topic). Let's now move onto the next tag on the agenda, the paragraph tag:
<!--hello_world.html-->
<h1>Hello World!</h1>
<p>This is some text meant to be part of a paragraph.</p>
In this simple example, we are presented with a heading tag, specifically an <h1>
tag, the "Hello World!" text acting as the heading, and then a "paragraph" or <p>
tag following it, with some rather overt text explaining that the text enclosed within it is a paragraph. If we now open up the file in the browser as before, we will see this simple example displayed:
As the caption of the above image suggests, the <h1>
and <p>
tags are considered "sibling" elements. This naming convention after members of a family is not a coincidence, and comes into play in both CSS and JavaScript (which utilizes the naming convention outright when referencing elements). Indeed, this convention is a good segue into the topic of the Document Object Model, abbreviated as the DOM. The topic of the DOM is of particular significance to Front End Development and is discussed further below, but before covering that topic, let us cover the very basics of nested HTML elements.
This next code snippet I will provide may indeed overwhelm those who are particularly new to the subject of Web Development and HTML, as it is much more extensive than the previous examples. Regardless, at some point or another it is important for anyone interested in learning Web Development to become familiar with the generic structure one finds in many HTML documents throughout the Web. Here is a basic template of what one starts off with when first writing a bare bones HTML document:
<!--hello_world.html-->
<!DOCTYPE html>
<html lang="en">
<head>
<title>My Hello World Example</title>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<link href="css/style.css" rel="stylesheet" />
</head>
<body>
<main>
<article>
<h1>Hello World!</h1>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod
tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim
veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea
commodo consequat. Duis aute irure dolor in reprehenderit in voluptate
velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint
occaecat cupidatat non proident, sunt in culpa qui officia deserunt
mollit anim id est laborum.
</p>
</article>
</main>
</body>
</html>
If you're new to HTML and are a bit overwhelmed by this above snippet, know that you're not alone, many who have accidentally opened up their browser's devtools by hitting the F12 key have often found themselves heavily intimidated by the vast lines of code. Even experienced developers will sometimes have eyes that glaze over at large bodies of text.
While there are some that would give you a slightly more gentle introduction to HTML, this particular example is a suitable base off of which to extend other examples in the following articles on CSS and JavaScript, and also gives us a good introduction to HTML nested elements ("child" and "parent" HTML elements). Let's break this document down in detail, starting with the first line:
<!--hello_world.html-->
To start off with, we have the first line which actually is commented out. This is for your reference as the reader to know that you are looking at, in this case, a file called "hello_world.html". This is actually not part of document, and the preceding lesser than symbol, "<", followed by the exclamation point and two dashes is meant to demarcate the beginning of an html "comment". After naming the file (hello_world.html), it is closed off by two dashes and a greater than symbol, ">". This means that when rendered, the browser will ignore and not display this bit of text. To reiterate, this bit of text is for your reference and is not the usual part of a standard HTML document. Let's move onto the next line:
<!DOCTYPE html>
This line may seem self explanatory, but is a convention from the early days of HTML, in which the Web Browser was told specifically what type of document it is being given to render. In this case, it is rendering an html document. Moving onto the next line, we encounter another relatively self explanatory line:
<html lang="en">
You probably can already understand this bit of logic, but to make it absolutely clear, the language this document is rendered in is english, using the commonly used prefix "en". This is also the overall "parent" tag of our document, appropriately named the <html>
tag. After this we encounter a <head>
tag, which encapsulates a series of tags of some interest, and introduces us to our first instance of nested elements. Let's break down these series of lines within the <head>
tag:
<!--hello_world.html-->
<!DOCTYPE html>
<html lang="en">
<head>
<title>My Hello World Example</title>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<link href="css/style.css" rel="stylesheet" />
</head>
I included the indentation here to introduce this concept of nesting first. I will cover what each of these tags mean in detail shortly. Recall our previous example where we had an <h1>
tag and a <p>
tag?:
<!--hello_world.html-->
<h1>Hello World!</h1>
<p>This is some text meant to be part of a paragraph.</p>
These are "sibling" HTML elements. They lie next to each other in the "flow" of the document and hold equivalent prevalence in the "hiearchy" of our document structure. While one sibling might be "older" than the other (the <h1>
element comes before the <p>
element in the document when reading from top to bottom), the text within then is easily recognized as being encapsulated by their closing tags, the </h1>
and </p>
tags specifically. In the more extensive example with the <head>
tag above, however, we see that there is specifically indentation, and other opening and closing tags can be seen within the content of the <head>
tag, specifically a <title>
tag, two <meta>
tags, and a <link>
tag. Additionally the <meta>
and <link>
tags don't appear to have a closing tag, but rather simply end with a forward slash at the end before the greater than symbol. So what exactly is happening here?
Well the first true tag of our document is the <html>
tag:
<html lang="en">
This tag starts our HTML document. In fact the closing tag for this tag is all the way at the very bottom of our document, meaning that all elements within the opening and closing html tags are "children" of this <html>
tag. Let's take a look at this introductory <header>
tag in its entirety once again:
<!--hello_world.html-->
<!DOCTYPE html>
<html lang="en">
<head>
<title>My Hello World Example</title>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<link href="css/style.css" rel="stylesheet" />
</head>
Similarly, we see that below our opening <head>
tag that the closing </head>
tag isn't encountered until four lines down, where it holds the same level of indentation (2 spaces specifically). Thusly, the title, two meta, and link tags are all "children" of the <head>
tag, which itself is a child of the <html>
tag. Note that the indentation is not what is demarcating this nesting convention, but rather the placement of where the closing tag for that opening tag occurs within the HTML document is how the Browser "determines" which elements are nested.
Each of the <title>
, two <meta>
, and <link>
tags are "siblings" to each other as they each are closed off, either by a traditional closing tag that you have seen before, as is the case with the title tag closing off with </title>
, or in the case of the <meta>
tags and <link>
tag, are closed off simply by appending a forward slash before the closing of the opening tag itself with the greater than symbol. This is another way of closing off certain tags in HTML documents, and is usually used for elements which hold no inner textual content.
Before moving onto the more extensive topic of the Document Object Model (DOM), I'd like to briefly cover what each of these elements are in turn, starting with the <head>
tag:
The <head>
tag is the very first child element of our HTML document, as it holds metadata (data about data) related to our document. It is a required tag and unless displaying an extremely basic text page, the HTML document will not render without this essential tag. Similary, the first child tag of the <head>
tag is also required, the <title>
tag. The text of this tag provides the title of the Web Page itself, and is what is rendered within the tab of the browser. In fact if we render this HTML document as we did before we will see the title, "My Hello World Example", rendered in the tab itself:
Following the <title>
tag, we are presented with our first <meta>
tag which delineates what character set the page is rendered in, in this case it is the UTF-8 standard. This is a character encoding standard that is an extensive topic on its own, and is beyond the scope of this article. If you wish to know more about UTF-8, please take a look at the corresponding wikipedia article.
Next we have the second <meta>
tag which contains metadata regarding the "viewport", "content", and "initial-scale". To summarize, this data is referencing how the browser will render the page based off of the initial width of the device, as well as the width of the browser. This meta tag is itself a good segue into what is known as "Responsive Web Design", which refers to how a web page is displayed depending on the screen size of the device it is being viewed on. I plan on covering more on responsive web design in my subsequent article on CSS, but for now if you're so inclined, please feel free to read the w3 schools article on the subect.
Lastly we have a <link>
tag, which includes an "href" attribute as well as a "rel" attribute. This <link>
tag in particular has a default value in its "href" attribute, which refers to a "style.css" file within the "css" directory. The "rel" attribute refers specifically to it being a "stylesheet". The "href" attribute contains a "url" or "Uniform Resource Locator". This is generally an address, either to a resource on the web, or a resource on the local device.
Finally the closing </head>
tag is encountered, and the metadata about our HTML document is complete. Let's now move onto the actual data of our article, starting with the <body>
tag:
<body>
<main>
<article>
<h1>Hello World!</h1>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod
tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim
veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea
commodo consequat. Duis aute irure dolor in reprehenderit in voluptate
velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint
occaecat cupidatat non proident, sunt in culpa qui officia deserunt
mollit anim id est laborum.
</p>
</article>
</main>
</body>
The <body>
tag is the meat of our document, including pretty much all content of our HTML document that is not metadata. This includes the nav and footer, article and paragraphs, as well as lists, tables, images, embedded video, and many other elements. While sibling to the <head>
tag, and child to the <html>
tag, it is usually the parent to pretty much every other element within the document.
Next we have the <main>
tag, which specifies the main content of the document. It might seem redundant to have a tag called "main" after the <body>
tag, but the <main>
tag is used primarily to differentiate the content of the HTML document from other elements used for navigation, like the nav and footer.
The following <article>
tag is used to delineate, according to the w3 schools article on the tag, a block of "independent, self-contained content". Generally this is used to identify content like a forum/blog post, a news story, or some other length of textual data. This is the child of the <main>
tag and parent to the remaining two tags, the <h1>
and <p>
tags.
We have already covered what the <h1>
and <p>
tags are in our original examples. I will briefly address the strange text you see within the <p>
tag as being some dummy text known as "lorem ipsum" text, which is generally used as a placeholder for a (usually large) body of text when the content of the article has yet to be written. This is used when the developer needs to have a visual representation of what the general text will look like in the document prior to publication.
This brief overview of the general flow of a standard HTML document is meant solely as an introduction to the concepts one will encounter when working as a Web Developer, specifically a Front End Web Developer. Having grasped the basic concepts of HTML tags, as well as sibling and child elements, let us now move onto the subject of the Document Object Model, more commonly referred to as the DOM:
The Document Object Model
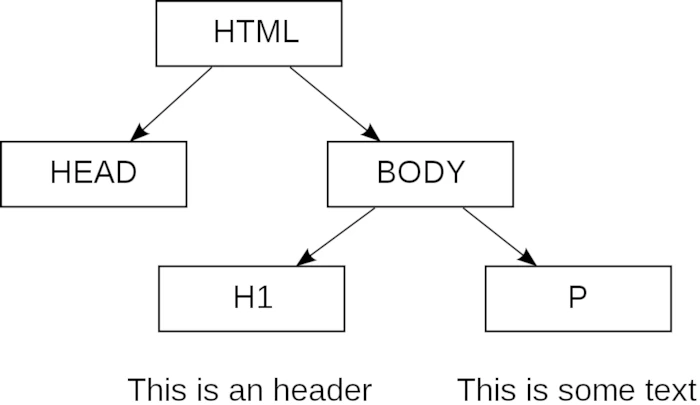
I'll be the first to admit that I'm still learning quite a bit about HTML, CSS, and JavaScript, as each are vast subjects in their own right that one could spend a lifetime trying to master. HTML, while unimpressive visually, provides a scaffolding onto which nearly any kind of two dimensional interface can be rendered and displayed back to the user. The Document Object Model (DOM) is more than just a simple abstraction when trying to conceptualize the structure and flow of an HTML document. Rather, the DOM is an interface through which every element in the HTML document is represented like branches and leaves on a tree.
These "nodes", as they are officially termed according to the W3C standards, can be interacted with via both CSS configuration and JavaScript programming. In understanding the structure of the DOM, we as Frontend Web Developers can stylize and manipulate the HTML document.
To review our previous subject on nested elements, let's take a look at a basic HTML structure:
<html>
<head>
<title>My Website</title>
</head>
<body>
<h1>Welcome</h1>
<p>This is my website.</p>
</body>
</html>
This can be represented textually in the DOM like so:
- Document (root)
- html
- head
- title
- "My Website"
-body
- h1
- "Welcome"
-p
- "This is my website."
Looking at our previous code snippet above, we can see that the "Document" is treated as the "root" node of the DOM. Very much like our tree analogy, this is the root of the HTML document, and is the first element parsed by the Browser when a GET request is made for the HTML web page. From here we have our familiar <html>
, <head>
, <title>
, <body>
, <h1>
, and <p>
tags all decending in order down the DOM tree. As referenced earlier, you can see that the <head>
and <body>
tags are siblings of each other, each with their own respective child elements. The textual content of the <title>
, <h1>
, and <p>
tags indicate that these nodes are the ending nodes in the tree, and are aptly named "leaf" nodes, as they are the farthest nodes from the root and have no children of their own.
The power of this structure is not obvious, especially if you have never interacted with the DOM via CSS or JavaScript before. In the following articles, it will hopefully become more apparent as to why HTML is structured in this fashion, and how one can start to make HTML sites more beautiful and interactive. Without CSS and JavaScript, websites, while informative, are little more than readable documents, occassionally with some links to other web pages. This is made apparent if you render the html document from our previous example in the browser:
Conclusion
In this article, I've made my best attempt to cover the basics of how a Webpage is brought to you over the Internet. First by delineating how a webpage is first requested from the user on the Frontend Client and then subsequently retrieved from the Backend Server. I then moved on to describe how the Browser interprets this requested data and renders it as HTML. This is done by interfacing with the Document Object Model (DOM), by which the Browser "traverses" its tree-like structure to eventually render the textual content of the page at its outermost "leaf" nodes.
If you've made it here, to the end of this article, I humbly thank you for taking the time to read this in its entirety. I know that the subject of pure HTML can appear rather bland even to the uninitiated, but I assure you that once we get more into the topics of CSS and JavaScript, that you will begin to understand why it was first necessary to dive into the basics of HTML first. The subtitle of this article refers to HTML as being "The Web's Skeleton." I named it this because this is the analogy I like best when thinking about Frontend Web Development in general. HTML is the skeleton on top of which we Web Developers can then add Makeup and Muscles.